Assignment 1: Pixel Processing Operations
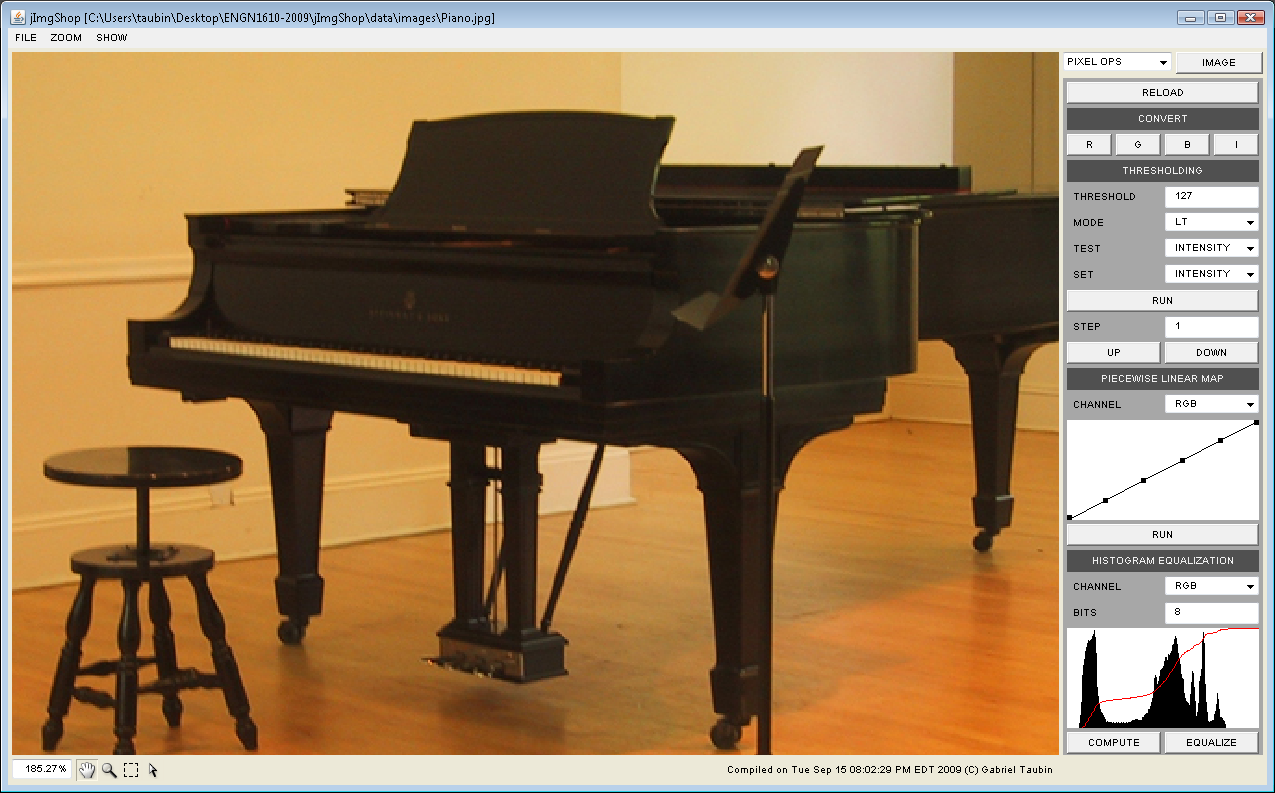
The goal of this assignment is to implement several linear and nonlinear image filters.
DATES
The assignment is out on Monday, September 10, 2012, and it is due on Friday, September 21, 2012.
ASSIGNMENT FILES
Download the zip file ENGN1610-2012-A1.zip containing all the Assignment 1 files. Unzip it to a location of your choice. You should have now a directory named assignment1 containing the following six files
- Makeflie
- JImgShopA1.zip
- JImgShopA1.lnk
- JImgShopA1.bash
- JImgPanelPixelOps.java
- ImgPixelOps.java
- docs
- images
- javac -O -classpath ".;JImgShopA1.zip" JImgPanelPixelOps.java
- JImgPanelPixelOps.class
- ImgPixelOps.class
In a windows machine you can run the JImgShop program by double clicking on the shortcut JImgShopA1.lnk. Otherwise, type the following command at a command promp.
- java -Xmx1000M -cp ".;jImgShopA1.zip" JImgShopApp -w 790 -h 800 -st
- java -Xmx1000M -cp ".:jImgShopA1.zip" JImgShopApp -w 790 -h 800 -st
The file JImgPanelPixelOps.java contains the implementation of the graphical user interface associated with this assignment. You don't need to modify this file unless you want to modify the design of the GUI.
The file ImgPixelOps.java contains the implementation of the class ImgPixelOps, which is designed to isolate the Pixel Processing Operations that you need to implement from the rest of the program. The class ImgPixelOps comprises the following public methods
- public Img convertToGray();
- public Img convertToRed();
- public Img convertToGreen();
- public Img convertToBlue();
- public Img threshold(int tMode, int tTest, int tSet, int value);
- public int[] histogramCompute(int nBits, int channel);
- public Img histogramEqualize(int nBits, int channel);
- public Img applyPwlMap(VecFloat pwlMap, int channel);
THE Img CLASS
In the subdirectory docs you will find a file named
- ImgPublic.java
- public int getWidth();
- public int getHeight();
- public int[] getPixel();
THE VecFloat CLASS
This class is used as an input parameter for the method applyPwlMap to represent a piecewice linear function from [0,1] into [0,1]. This class is a container for a variable length array of float variables. In the subdirectory docs you will also find a file named
- VecFloatPublic.java
- public int size();
- public float get(int i);
THE ImgPixelOps CLASS
For this assignment you need to complete the implementation of this class.
public Img convertToGray()
This method is fully implemented as an example.
public Img convertToRed()
You need to make each pixel red by setting the green and blue components to zero.
public Img convertToGreen()
You need to make each pixel green by setting the red and blue components to zero.
public Img convertToBlue()
You need to make each pixel blue by setting the red and green components to zero.
public Img threshold(int tMode, int tTest, int tSet, int value)
You need to test the image component specified by the tTest component of the image against the threshold value, and modify the pixel value component specified by the tSet variable. The variable tMod specifies what kind of comparison to make. The details are provided as comments in the provided ImgPixelOps.java file.
public int[] histogramCompute(int nBits, int channel)
You need to compute a histogram of length 1<
public Img histogramEqualize(int nBits, int channel)
You need to histogram equalize the image component specified by the channel variable. If the RGB component is specified, then you need to histogram equalize the red green, and bluee components independently of each other.
public Img applyPwlMap(VecFloat pwlMap, int channel)
You need to map the image component specified by the channel variable onto itself, using the piecewise linear function specified in the pwlMap variable. The piecewise linear function is specified by nKeys = pwlMap.size()/2 (key,value) pairs, which can be accessed as follows
- float key = pwlMap.get(2*i );
- float value = pwlMap.get(2*i+1);
WHAT TO SUBMIT
You need to submit your edited file ImgPixelOps.java. Make sure that it is well commented out. Explain all your choices in the comments. If you were not able to make somethig work, explain the problems here. If you modify the user interface, then you also need to submit your edited JImgPanelPixelOps.java file. If you need to explain things with formulas, you can also send us a document. Create a zip file named ENGN1610-A1-NAME.zip and send it to the instructor as an attachment, where NAME is your last name.